One of the harder imports that you may have to do in The Raiser’s Edge is importing recurring gifts with schedules. The regular help file itself suggests itself that it is tricky but then unfortunately does not offer any examples. Writing code to create various schedules is equally as difficult if not harder. This post does apply to pledges with schedules but, here in the UK, recurring gifts are much more common than pledges with schedules so I will concentrate on that but the same principles apply. I have also noticed that there are more and more people developing in C# so this is for them (but is not that hard to translate to VB.NET)
It is well documented how you create a gift and how you put all the values on those fields. Here is our basic framework for the examples in this article:
private void CreateGift(IBBSessionContext session, int constitId) { CGift gift = null; try { gift = new CGiftClass(); gift.Init(ref session); gift.set_Fields(EGiftFields.GIFT_fld_Constit_ID, constitId); gift.set_Fields(EGiftFields.GIFT_fld_Type, "Recurring Gift"); gift.set_Fields(EGiftFields.GIFT_fld_Date, "05/08/2009"); gift.set_Fields(EGiftFields.GIFT_fld_Amount, 10); gift.set_Fields(EGiftFields.GIFT_fld_Fund, "General Fund"; gift.set_Fields(EGiftFields.GIFT_fld_Campaign, "2009 Campaign"); gift.set_Fields(EGiftFields.GIFT_fld_Appeal, "Phone-a-thon 2009"); gift.Save();
} catch (Exception e) { throw e; } finally { if (gift != null) { gift.CloseDown(); System.Runtime.InteropServices.Marshal.ReleaseComObject(gift); System.Runtime.InteropServices.Marshal.FinalReleaseComObject(gift); gift = null; } } }
Now we need to add on the schedule.
First for a monthly payment. There are two methods; either a day of the month or an ordinal day of the week e.g. The 1st Wednesday of every 1 month. The code below shows the former:
gift.set_Fields(EGiftFields.GIFT_fld_Installment_Frequency, "Monthly"); gift.set_Fields(EGiftFields.GIFT_fld_Schedule_Spacing, 1); gift.set_Fields(EGiftFields.GIFT_fld_Schedule_DayOfMonth, 31); gift.set_Fields(EGiftFields.GIFT_fld_Schedule_MonthlyType, "Specific day"); gift.set_Fields(EGiftFields.GIFT_fld_Date_1st_Pay, "6/10/2009");
Now for an annual gift:
gift.set_Fields(EGiftFields.GIFT_fld_Installment_Frequency, "Annually"); gift.set_Fields(EGiftFields.GIFT_fld_Schedule_Spacing, 1); gift.set_Fields(EGiftFields.GIFT_fld_Schedule_DayOfMonth, memDet.FirstScheduledDate.Value.Day); gift.set_Fields(EGiftFields.GIFT_fld_Schedule_Month, System.Threading.Thread.CurrentThread.CurrentCulture.DateTimeFormat.GetMonthName(5)); gift.set_Fields(EGiftFields.GIFT_fld_Schedule_MonthlyType, "Specific day"); gift.set_Fields(EGiftFields.GIFT_fld_Date_1st_Pay, "6/10/2009");
The annual gift is very similar to monthly only that we have to add the month of the year. Here I am using the DateTimeFormat object to get the localised month name for May. However if you are in a non-English speaking country then this will not work with RE and you will want to either hard code the values or set the culture to en-US, en-GB, etc so that it pulls “May” and not local version of that month.
The diagrams below show the different values and how they are used. Note that the monthly diagram has been photoshopped to show both the ordinal and the day of the month schedules at the same time. From this you can see it is relatively simple to create any permutation of schedule.
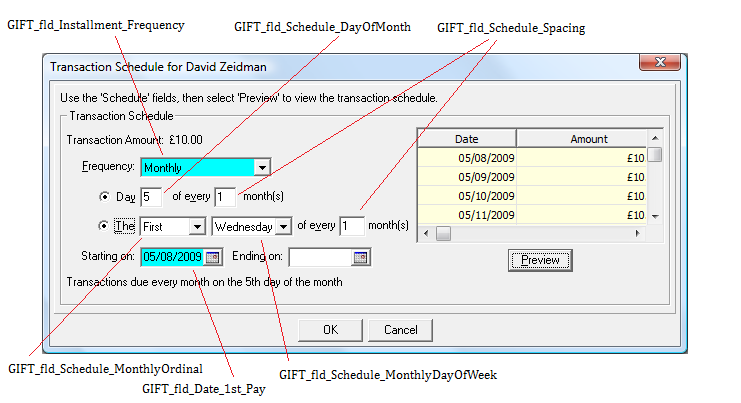
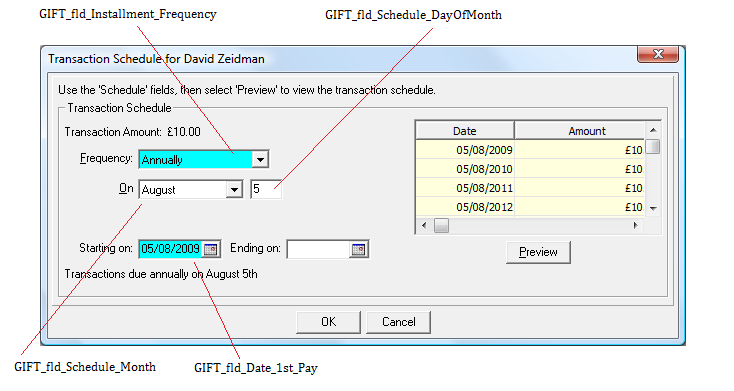